Integrate with a new channel
In this tutorial, you will walk through the process of building an integration that will allow Gorgias users to receive and respond to tickets from a generic SMS application.
Step 1: Create an endpoint to receive data from Gorgias
Gorgias can send out HTTP requests when a ticket is created, updated, or another ticket message is added to the conversation. To receive information from tickets and new messages sent from Gorgias, you will have to create an endpoint for the HTTP integration to call.
Step 2: Have your user set up an HTTP integration in Gorgias
On Gorgias, go to the HTTP Integration page: Gorgias -> Settings -> Integrations -> HTTP Integrations and select the "Create integration button"
Have your user input the endpoint from step 1 as the URL.
Step 3: Retrieve a full list of customers from your client's Gorgias account
When a customer sends an SMS to a client, you will have to create a ticket. Before creating a ticket in Gorgias, you will need to either create or update a customer with their SMS channel information.
You can grab all customers on a Gorgias account through the following request:
curl --request Get \
--url https://artemisathletix.gorgias.com/api/customers/ \
--header 'accept: application/json' \
--header 'authorization: Basic {username:password_encodedinbit64}' \
--header 'content-type: application/json' \
This request will return a response in the form of a list of all customers in the account, paginated.
{
"data": [{
"id": 281946293,
"external_id": null,
"active": true,
"email": "accounts@1password.com",
"name": "1Password",
"firstname": "1Password",
"lastname": "",
"language": null,
"timezone": null,
"created_datetime": "2020-01-07T02:37:52.244681+00:00",
"updated_datetime": "2020-01-07T02:37:52.247977+00:00",
"meta": {
"name_set_via": "email"
},
"note": null
},
{
"id": 314516961,
"external_id": null,
"active": true,
"email": "bobsmith@gmail.com",
"name": "Bob Smith",
"firstname": "Bob",
"lastname": "Smith",
"language": null,
"timezone": null,
"created_datetime": "2020-03-09T19:53:45.367945+00:00",
"updated_datetime": "2020-03-09T21:00:46.466988+00:00",
"meta": {
"name_set_via": "email"
},
"note": null
}
],
"object": "list",
"uri": "/api/customers/?",
"meta": {
"page": 1,
"per_page": 30,
"current_page": "/api/customers/?page=1",
"item_count": 901,
"nb_pages": 31,
"next_page": "/api/customers/?page=2"
}
}
You can learn more about the Customer object in our API documentation .
Step 4: Create or update the customer in Gorgias
In Gorgias, every Customer object has a UserChannel object, which holds the addresses to communicate with a user.
If a user does not exist in Gorgias, you can create a customer by making a POST call to the api/customers/ endpoint. You can populate this user with the information available in the third-party application.
curl --request POST 'https://artemisathletix.gorgias.com/api/customers' \
--header 'Content-Type: application/json' \
--header 'Authorization: Basic {username:password_encodedinbit64}' \
--data '{
"name": "Bob Smith"
"note":"",
"channels":[
{
"address":"+13061111111",
"type":"phone"
}
]
}'
If the customer already exists without an SMS channel, you must update a customer's with channels via a PUT call to the api/customers/ endpoint, like so:
curl --request PUT 'https://artemisathletix.gorgias.com/api/customers' \
--header 'Content-Type: application/json' \
--header 'Authorization: Basic {username:password_encodedinbit64}' \
--data '{
"channels":[
{
"address":"bobsmith@gmail.com",
"type":"email"
},
{
"address":+13061111111,
"type":"phone"
}
]
}'
Step 5: Create a ticket linked to the Gorgias user
Once your customer has been created or updated, you should create a ticket in Gorgias populated with the first message via a POST call.
For SMS Integrations, Gorgias users can only respond via email channels, which can be used to trigger an SMS response from the third-party platform's API.
Therefore, when responding to messages, the ticket's sender should be set as an email with an address like "smsintegration+{phonenumber}@externalservice.com.
curl --location --request POST 'https://artemisathletix.gorgias.com/api/tickets/' \
--header 'Content-Type: application/json' \
--header 'Authorization: Basic {username:password_encodedinbit64}' \
--data: '{
"messages": [
{
"channel": "phone",
"via": "api",
"from_agent": false,
"sender": {
"email": "smsintegration+{phonenumber}@externalservice.com",
}
"receiver": {
"email": "support@gorgias.com"
},
"subject": "Ticket created via API",
"body_text": "Hi, this is a ticket created from the API.",
"body_html": "<div>Hi, this is a ticket created from the API.</div>",
"from_agent": true
}
}
]
}'
This request will generate a ticket in the app, which you use to reply to the customer via email.
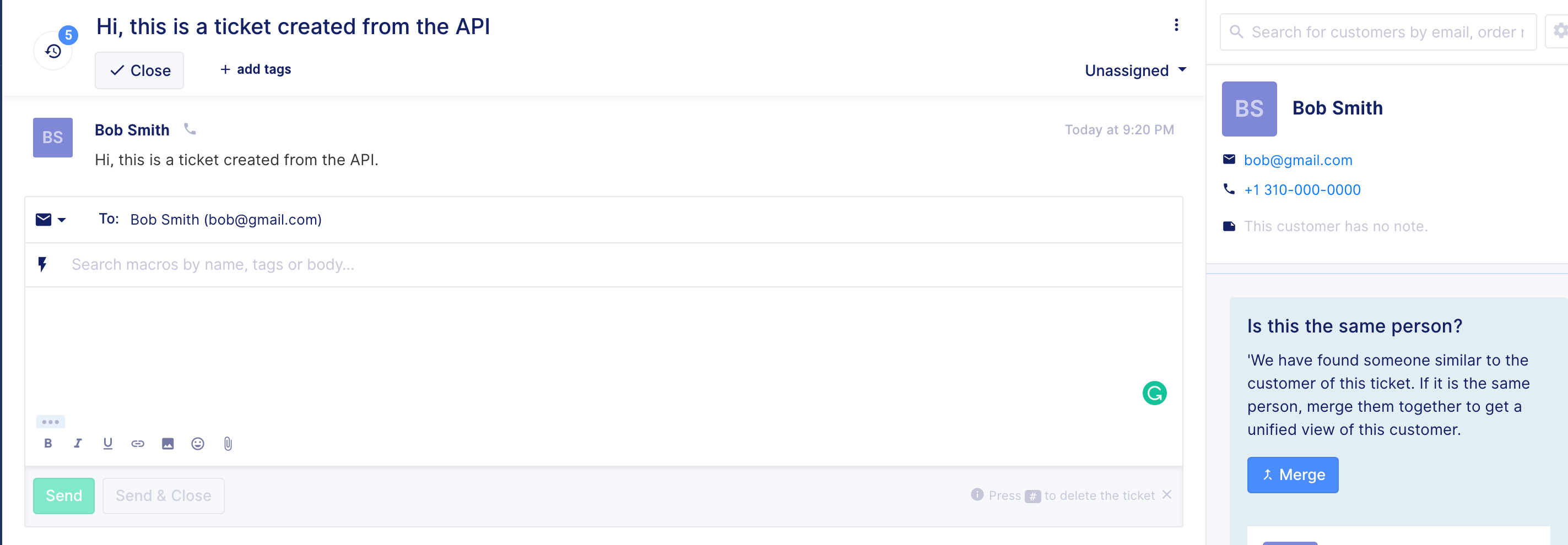
Step 6 - Send SMS from third-party platform
After a Gorgias user has responded to a ticket message via SMS, the HTTP integration should send a webhook from Gorgias with the message information from
When receiving the new message should smsintegration+{phonenumber}@externalservice.com, the external service should forward an SMS to the {phonenumber}, using the third party application's API.
This will allow you to respond to customers on Gorgias' platform.
Updated over 2 years ago